Getting started with Gremlin’s Python SDK
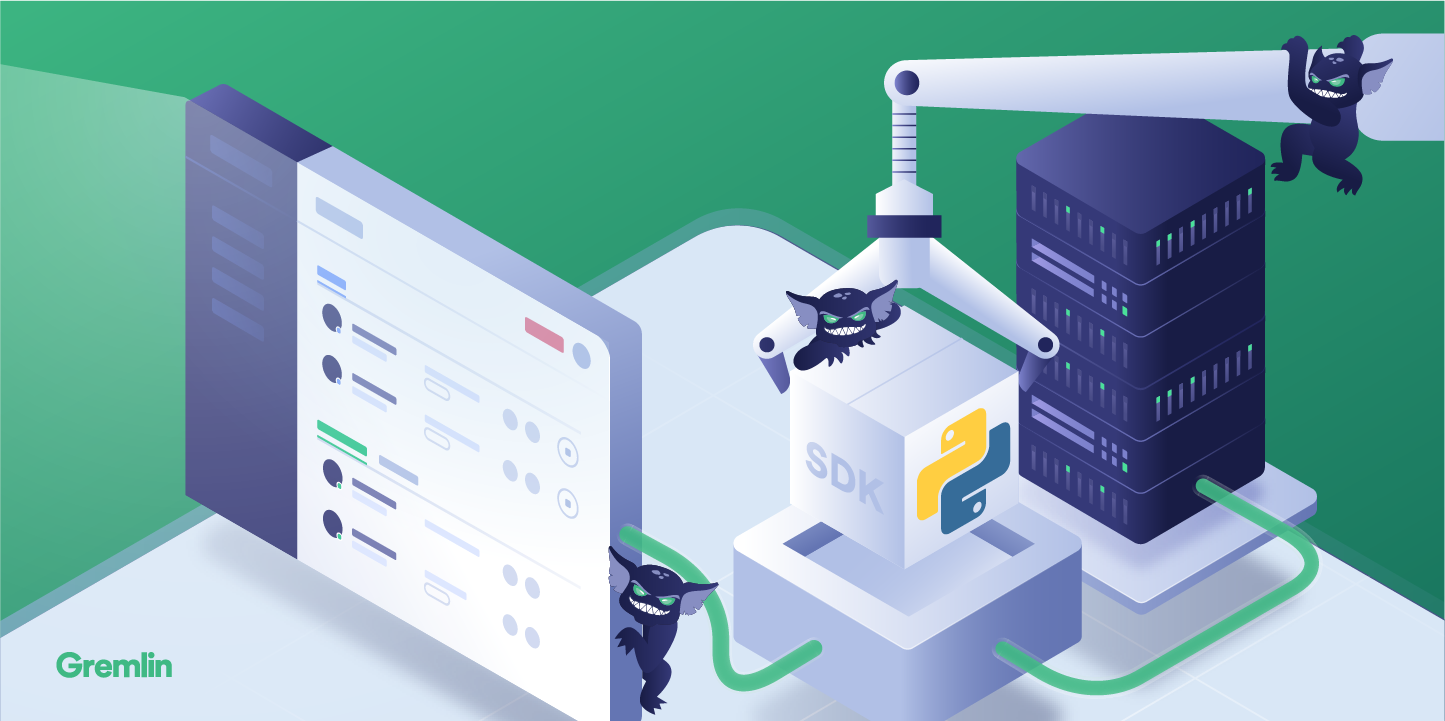
Introduction
Using the Gremlin UI is an easy, visual way to run Chaos Experiments. But as your applications become more reliable and able to withstand attacks, automating your Chaos will prevent you from drifting back into failure. This tutorial will get you started using Gremlin's Python SDK for the Gremlin API.
Prerequisites
To successfully complete this course, you’ll need:
- A Repl.it Account - Repl.it is an online tool that allows you collaboratively write and run code. This will be provided by your Bootcamp Manager
- A Gremlin account. This will be provided by your Bootcamp Manager as well.
- A Datadog account. This will be provided by your Bootcamp Manager as well.
Overview
The Gremlin Python SDK makes it easy to interact with Gremin's API. You can use it to get information about your account, launch attacks and see their status, create scenarios, and more. In this tutorial we'll be using the SDK to connect to Gremlin, launch an attack, get its status and return the results when it's finished.
Step 0
Log in to Repl.it, Gremlin, and Datadog with the credentials provided.
Step 1
After you've logged into Repl.it, click the "My Repls" link in the left side navigation, then open the Gremlin Python SDK project.
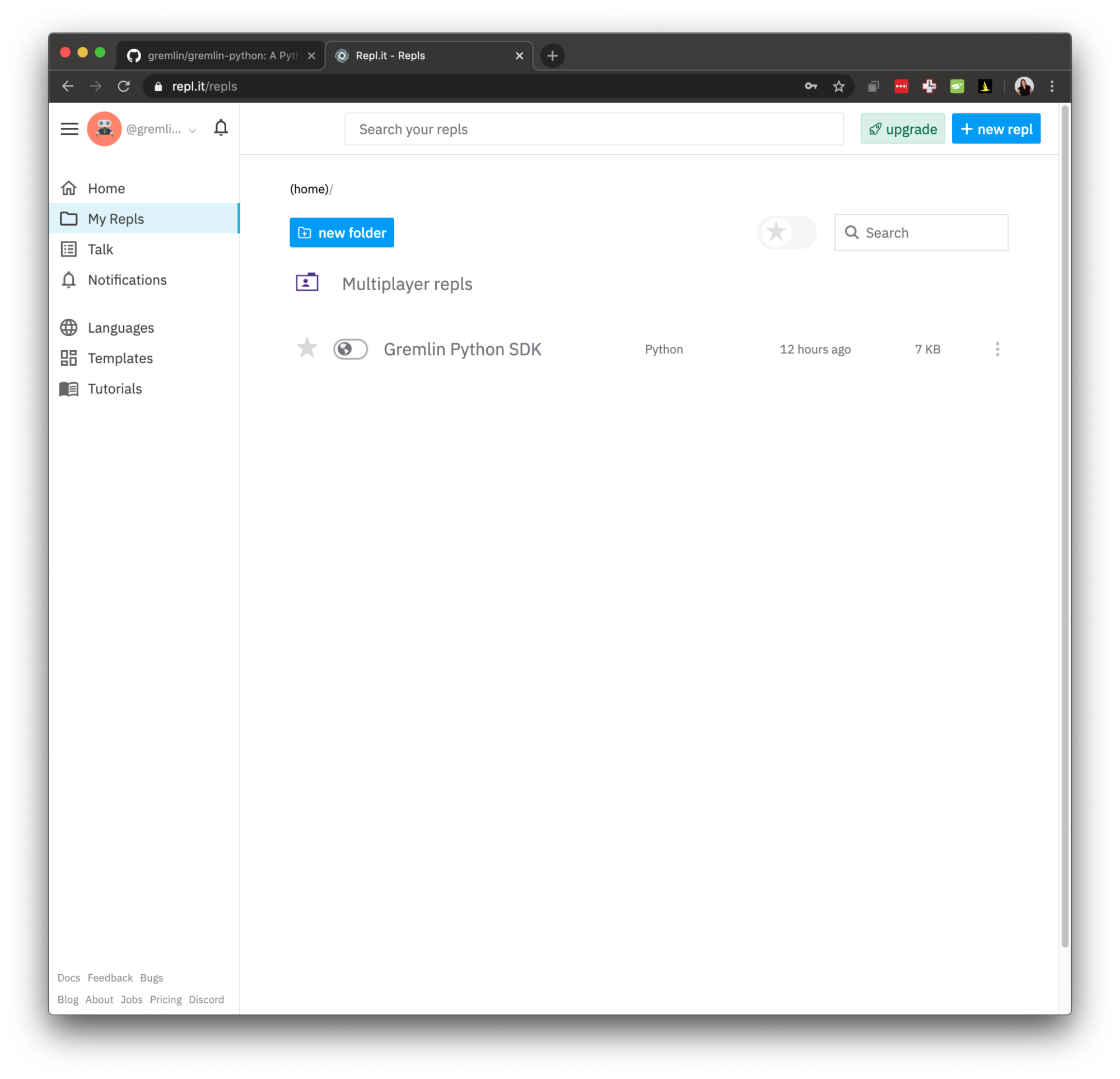
The project has already loaded the <span class="code-class-custom">gremlinapi</span> python library and the code has imported the the modules that we'll be using for the tutorial.

To connect to the Gremlin API, you'll first need to set your Gremlin Team ID. In the Gremlin application, go to your "Team Settings", then visit "Configuration", and copy your Team ID and paste it below. For example:
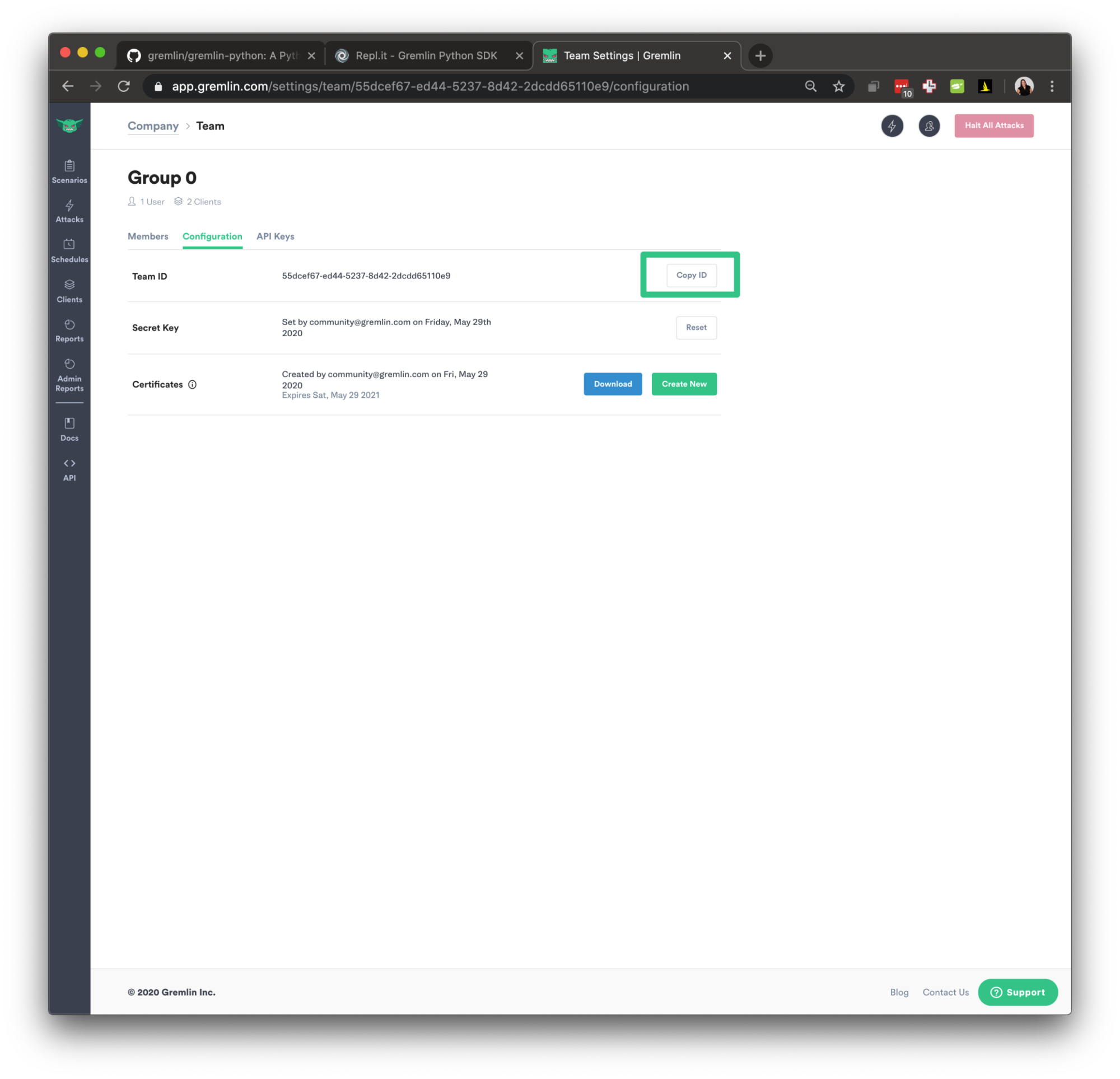
Step 2
You'll also need an API key to connect to Gremlin. In the Gremlin application, go to your "Account Settings", then visit "API Keys", and create an API Key in the Gremlin application and paste it below.
Copy your API Key and paste it into the code. Note that you can easily copy the api key by clicking the eye icon next to the API Key name, then clicking the "Click to copy" message that appears.
After you've copied your Team ID and API Key, click the "run" button.
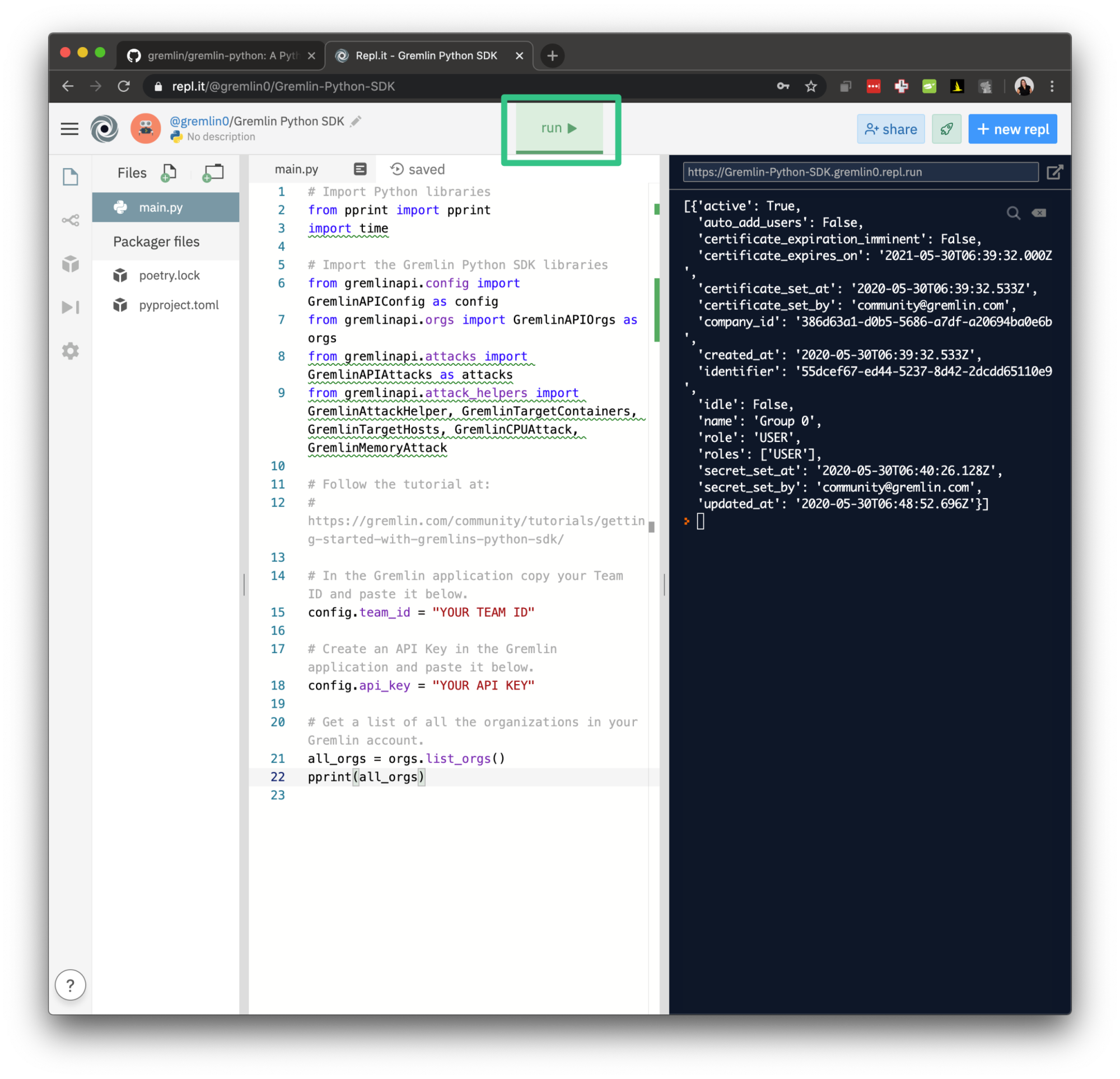
Your application will user your credentials to connect to the Gremlin API to retrieve a list of your Gremlin organization and print the results.

Step 3
Next we'll run an attack by using the <span class="code-class-custom">attacks.create_attack()</span> method. We'll also use the <span class="code-class-custom">GremlinAttackHelper()</span> to easily format our attack parameters. Copy and paste the following code into your application. This will launch a memory attack of 100% of yours as defined by <span class="code-class-custom">GremlinTargetHosts</span>. The memory attack will run for a length of 240 seconds and inject an increase of 85%
Run your application to launch the attack. Click "Attacks in the left side navigation in Gremlin to view your currently running attacks and you'll see the attack you just created via code!
Among your team discuss:
- Compared to my hypothesis, how is my application handling this failure?
- Are we able to notice anything on Datadog?
- What can we do to make our application more resilient to conditions like this?
Step 4
Although the Gremlin application is a great way to visually see what attacks are running, we need to be able to verify the attack via code and report its status.
To do this, we'll create a simple loop that polls the Gremlin attack status every 5 seconds by calling the <span class="code-class-custom">attack.get_attack()</span> method.
Copy and paste the following code into your application:
Run your application to launch the attack again and see the attack status via the application.
Example Code
If you would like to see it all working, this is an example implementation you can copy and paste into your application.
Next Steps
This tutorial provides a brief look at how you can run an attack and get the attack status using the Gremlin Python SDK. To take your learning further, try changing the attack parameters or launching a different attack. You can find more information in the Gremlin Python SDK repository README file and in the Examples directory.
Conclusion
By using the Gremlin Python SDK, you can programmatically interact with the Gremlin platform. This provides an easy way to automate your Chaos Engineering practices.
Avoid downtime. Use Gremlin to turn failure into resilience.
Gremlin empowers you to proactively root out failure before it causes downtime. See how you can harness chaos to build resilient systems by requesting a demo of Gremlin.
